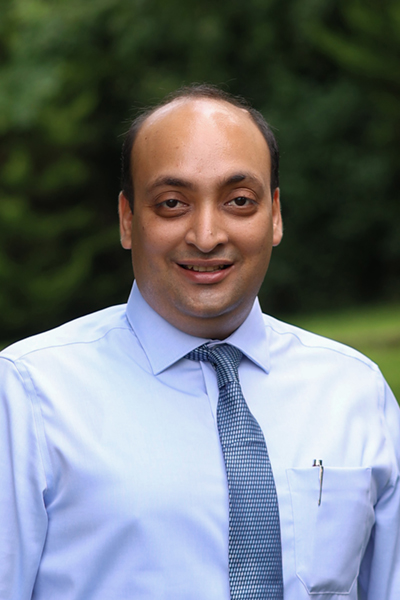
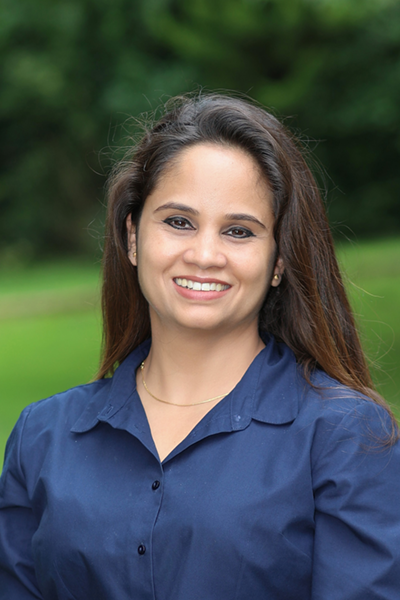
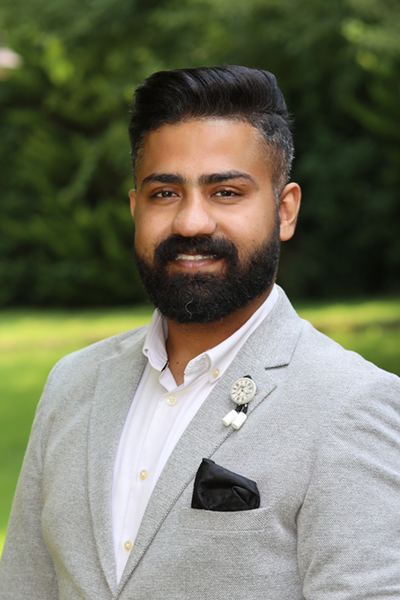
# This program prints Hello, world! print('Hello, world!')
# This program adds two numbers num1 = 1.5 num2 = 6.3 # Add two numbers sum = num1 + num2 # Display the sum print('The sum of num1 and num2 is ',sum)
# Store input numbers num1 = input('Enter first number: ') num2 = input('Enter second number: ') # Add two numbers sum = float(num1) + float(num2) # Display the sum print('The sum of num1 and num2 is ',sum)
a = float(input('Enter first side: ')) b = float(input('Enter second side: ')) c = float(input('Enter third side: ')) s = (a + b + c) / 2 area = (s*(s-a)*(s-b)*(s-c)) ** 0.5 print('The area of the triangle is ' ,area)
x = input('Enter value of x: ') y = input('Enter value of y: ') print('The value of x before swapping: ',x) print('The value of y before swapping: ',y) temp = x x = y y = temp print('The value of x after swapping: ',x) print('The value of y after swapping: ',y)
print("Enter the Principle Amount: ") p = int(input()) print("Enter Rate of Interest (%): ") r = float(input()) print("Enter Time Period: ") t = float(input()) si = (p*r*t)/100 print("Simple Interest Amount: ") print(si)
pi = 3.14 diameter = int(input("enter the diameter of the circle")) radius = diameter / 2 perimeter = 2 * pi * radius print("perimeter of circle is ", perimeter)
num = int(input("enter the number : ")) if(num%2==0): print("It is Even number") else: print("It is Odd number")
age = int(input("enter the age : ")) if(age>=18): print("You can vote") else: print("You can't vote")
pi = 3.14 radius = int(input("enter the radius of the circle")) area = pi * radius * radius print("area of circle is ", area)
kilometers = float(input("Enter value in kilometers: ")) conv_fac = 0.621371 miles = kilometers * conv_fac print("kilometers is equal to miles",miles))
b=float(input("Enter the base of triangle")) h=float(input("Enter the height of triangle")) Area=(1/2)*b*h print("The area of triangle is : ", Area)
a = float(input("Enter the dividend : ")) b = float(input("Enter the divisor : ")) Q = a // b R = a % b print("The quotient is : ", Q) print('The remainder is : ", R)
a=int(input('Enter the first integer:')) b=int(input('Enter the second integer:')) c=int(input('Enter the third integer:')) if a>b and a>c: print(a, 'is the largest integer') elif b>c: print(b, 'is the largest integer') else: print(c, 'is the largest integer')
a=float(input('Enter the first number:')) b=float(input('Enter the second number:')) if a%b==0: print('The first number is fully divisible by second number') else: print('The first number is not fully divisible by second number')
ticket_price = 20 # price per ticket num_tickets = int(input("Enter the number of tickets to buy: ")) if num_tickets <= 10: discount = 0.1 # 10% discount for 10 or fewer tickets elif num_tickets <= 20: discount = 0.2 # 20% discount for 11 to 20 tickets else: print("Maximum of 20 tickets allowed per transaction") total_cost = None if num_tickets <= 20: total_cost = num_tickets * ticket_price * (1 - discount) if total_cost is not None: print("Total cost for tickets: ",total_cost)
num_students = 600 subjects = ['Maths', 'Science', 'English', 'IT'] marks = {subject: [] for subject in subjects} # Input marks for each subject for all students for subject in subjects: print(f"Enter {subject} marks for {num_students} students:") for i in range(num_students): mark = float(input(f"Enter mark for student {i + 1}: ")) marks[subject].append(mark) # Initialize variables for highest and lowest marks overall_highest = -1 overall_lowest = 101 # Calculate highest, lowest, and average marks for each subject for subject in subjects: subject_marks = marks[subject] highest = max(subject_marks) lowest = min(subject_marks) average = sum(subject_marks) / num_students print(f"{subject} - Highest: {highest}, Lowest: {lowest}, Average: {average:.2f}") # Update overall highest and lowest marks overall_highest = max(overall_highest, highest) overall_lowest = min(overall_lowest, lowest) # Calculate overall average mark all_marks = [mark for sublist in marks.values() for mark in sublist] overall_average = sum(all_marks) / (num_students * len(subjects)) print(f"nOverall - Highest: {overall_highest}, Lowest: {overall_lowest}, Average: {overall_average:.2f}")
Regular Buyer = 10% discount
Item Name Units Country Tax (per unit)
Laptop (650 USD) 1 – 10 UK 10 USD
Laptop (700 USD) 11 – 30 UK 8 USD
Laptop (900 USD) 1 – 10 US 20 USD
Laptop (850 USD) 11 – 30 US 17 USD
print("1: UK") print("2: US") country = int(input("enter your choice : ")) if(country==1): units = int(input("enter units : ")) if(units<=10): price = ((units*650) + (units*10)) print("You have to pay : ",price) elif(units<=30): price = ((units*700) + (units*8)) print("You have to pay : ",price) else: print("you can't enter more than 30") elif(country==2): units = int(input("enter units : ")) if(units<=10): price = ((units*900) + (units*20)) print("You have to pay : ",price) elif(units<=30): price = ((units*850) + (units*17)) print("You have to pay : ",price) else: print("you can't enter more than 30") else: print("not a valid country") print("1: Regular Buyer") print("2: Not a Regular Buyer") choice = int(input("are you a regular buyer or not? : ")) if(choice==1): dis = price * 0.90 print("Price after discount = ",dis) else: print("No discount please pay= ",price)
Coupon Code: //Ask at the time of booking
Room Type: //Ask at the time of booking
Maximum: 2 Adults and 2 children in 1 room
print("1: Executive Suite") print("2: Delux Suite") print("3: Standard Room") choice = int(input("enter room type ")) if(choice==1): adults = int(input("enter no of adults ")) childrens = int(input("enter no of childrens ")) if(adults>2 or childrens>2): print("members exceeding") else: price = adults * 300 + childrens * 200 print("Please Pay : ",price) elif(choice==2): adults = int(input("enter no of adults ")) childrens = int(input("enter no of childrens ")) if(adults>2 or childrens>2): print("members exceeding") else: price = adults * 200 + childrens * 100 print("Please Pay : ",price) elif(choice==3): adults = int(input("enter no of adults ")) childrens = int(input("enter no of childrens ")) if(adults>2 or childrens>2): print("members exceeding") else: price = adults * 100 + childrens * 50 print("Please Pay : ",price) else: print("this room type is not available") print("Do you have a coupon?") print("1: Yes") print("2: No") c = int(input("enter your choice ")) if(c==1): coupon = int(input("enter the code")) if(coupon==545): discountedprice = price*0.80 print("Coupon Applied - please pay : ",discountedprice) elif(coupon==543): discountedprice = price*0.90 print("Coupon Applied - please pay : ",discountedprice) else: print("Invalid Coupon") else: print("No Discount Please Pay : ",price)
Currency Convert To Rate Agent / Bank
USD TL 18.60 10% , 12%
GBP TL 21.92 5% , 2%
Euro TL 19.22 8% , 5%
print("1: USD to TL") print("2: GBP TO TL") print("3: Euro to TL") choice = int(input("choose currency ")) if(choice==1): usd = int(input("how much USD?")) tl = usd * 18.60 agentc = tl * 0.10 bankc = tl * 0.12 print("Bank will give ",round(tl-bankc)) print("Agent will give ",round(tl-agentc)) if(agentc<bankc): print("Agent preferred") else: print("Bank preferred") elif(choice==2): usd = int(input("how much USD?")) tl = usd * 21.92 agentc = tl * 0.05 bankc = tl * 0.02 print("Bank will give ",round(tl-bankc)) print("Agent will give ",round(tl-agentc)) if(agentc<bankc): print("Agent preferred") else: print("Bank preferred") elif(choice==3): usd = int(input("how much USD?")) tl = usd * 19.22 agentc = tl * 0.08 bankc = tl * 0.05 print("Bank will give ",round(tl-bankc)) print("Agent will give ",round(tl-agentc)) if(agentc<bankc): print("Agent preferred") else: print("Bank preferred") else: print("Invalid currency selection")
Allow users the select the MacBook model and customize it as per the:
1. MacBook Air:
2. MacBook Pro:
price=0 print("1: Macbook Air") print("2: Macbook Pro") choice = int(input("select the product :")) if(choice==1): print("1: 8GB") print("2: 16GB +400$") print("3: 32GB +800$") ram = int(input("Select: RAM for Macbook Air")) if(ram==1): priceram = 0 elif(ram==2): priceram = 400 elif(ram==3): priceram = 800 else: print("not a RAM option") print("1: 256GB") print("2: 512GB +800$") print("3: 1TB +1600$") ssd = int(input("Select: SSD for Macbook Air")) if(ssd==1): pricessd = 0 elif(ssd==2): pricessd = 800 elif(ssd==3): pricessd = 1600 else: print("not a SSD option") totalprice = 2000 + priceram + pricessd print("Total Price of Macbook Air is: ",totalprice) if(choice==2): print("1: 8GB") print("2: 16GB +500$") print("3: 32GB +1000$") ram = int(input("Select: RAM for Macbook Pro")) if(ram==1): priceram = 0 elif(ram==2): priceram = 400 elif(ram==3): priceram = 800 else: print("not a RAM option") print("1: 256GB") print("2: 512GB +800$") print("3: 1TB +1200$") ssd = int(input("Select: SSD for Macbook Pro")) if(ssd==1): pricessd = 0 elif(ssd==2): pricessd = 800 elif(ssd==3): pricessd = 1200 else: print("not a SSD option") totalprice = 2500 + priceram + pricessd print("Total Price of Macbook Pro is: ",totalprice)
# writing to and reading a line of text from a file MyFile = open ("MyText.txt","w") TextLine = input("Please enter a line of text ") MyFile.write(TextLine) MyFile.close() print("The file contains this line of text") MyFile = open ("MyText.txt","r") TextLine = MyFile.read() print(TextLine) MyFile.close()
Pseudocode
DECLARE TextLine : STRING // variables are declared as normal
DECLARE MyFile : STRING
MyFile ← “MyText.txt”
// writing the line of text to the file
OPEN MyFile FOR WRITE // opens file for writing
OUTPUT “Please enter a line of text”
INPUT TextLine
WRITEFILE, TextLine // writes a line of text to the file
CLOSEFILE(MyFile) // closes the file// reading the line of text from the file
OUTPUT “The file contains this line of text:”
OPEN MyFile FOR READ // opens file for reading
READFILE, TextLine // reads a line of text from the file
OUTPUT TextLine
CLOSEFILE(MyFile) // closes the file
nums = [] length = int(input("Type length of list: ")) for x in range(length): numberadd = int(input("Type any number to add to list: ")) nums.append(numberadd) print(nums) number = int(input("type the number you want to find in list: ")) count = 0 while True: count=count+1 if nums[count] == number: break else: continue print("The number: ",number,"is in place: ",count+1)
bank_acount_check = int(input("Type the last 4 digits of your phone number to access your bank acount: ")) if bank_acount_check == 4444: balance = 1000 while True: def deposit(d,balance): print("You have deposited: ",d,". You have: ",balance,"USD in your bank acount balance") def withdraw(w,balance): print("You have withdrawn: ",w,"from your bank acount balance. You have: ",balance,"USD left in your bank acount balance") if balance < 0: print("Your are in debt") elif balance == 0: print("Your are bank rupt") def display(balance): print("Your bank acount balance has: ",balance,"USD") c = input("Type d to deposit | Type w to withdraw | Type display to display | Type close to close bank acount: ") if c == "d": d = int(input("Type how much to deposit: ")) balance=balance+d deposit(d,balance) elif c == "w": w = int(input("Type how much to withdraw: ")) balance=balance-w withdraw(w,balance) elif c == "display": display(balance) elif c == "close": break else: print("ERROR: Invalid option") print("You have closed your bank acount") else: print("ERROR: Invalid last 4 digits of your phone number, you were not able to access your bank acount")
# Python program to demonstrate printing # of complete multidimensional list a = [[2, 4, 6, 8, 10], [3, 6, 9, 12, 15], [4, 8, 12, 16, 20]] print(a)
# Python program to demonstrate printing # of complete multidimensional list row # by row. a = [[2, 4, 6, 8, 10], [3, 6, 9, 12, 15], [4, 8, 12, 16, 20]] for record in a: print(record)
# Python program to demonstrate that we # can access multidimensional list using # square brackets a = [ [2, 4, 6, 8 ], [ 1, 3, 5, 7 ], [ 8, 6, 4, 2 ], [ 7, 5, 3, 1 ] ] for i in range(len(a)) : for j in range(len(a[i])) : print(a[i][j], end=" ") print()
# Adding a sublist at the end a = [[2, 4, 6, 8, 10], [3, 6, 9, 12, 15], [4, 8, 12, 16, 20]] a.append([5, 10, 15, 20, 25]) print(a)
# Extending a sublist first row a = [[2, 4, 6, 8, 10], [3, 6, 9, 12, 15], [4, 8, 12, 16, 20]] a[0].extend([12, 14, 16, 18]) print(a)
# Initialize a 2D array array_2d = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] # Print elements of the 2D array for row in array_2d: for elem in row: print(elem, end=" ") print() # Move to the next line after printing each row
# Initialize a 2D array array_2d = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] # Find the sum of elements array_sum = 0 for row in array_2d: for elem in row: array_sum += elem print("Sum of elements:", array_sum)
# Initialize a 2D array array_2d = [ [1, 2, 3], [4, 5, 6], [7, 8, 9] ] # Print elements of the 2D array using row and column indices for i in range(len(array_2d)): for j in range(len(array_2d[i])): print(array_2d[i][j], end=" ") print() # Move to the next line after printing each row
# Function to calculate grade def calculate_grade(average_mark): if average_mark >= 70: return "distinction" elif average_mark >= 55: return "merit" elif average_mark >= 40: return "pass" else: return "fail" # Example data StudentName = ["Alice", "Bob", "Charlie"] StudentMark = [ [80, 75, 90], # Alice's marks for each subject [65, 70, 75], # Bob's marks for each subject [40, 55, 35] # Charlie's marks for each subject ] # Iterate over each student for i in range(len(StudentName)): total_mark = sum(StudentMark[i]) # Calculate total mark for the student average_mark = round(total_mark / len(StudentMark[i])) # Calculate average mark grade = calculate_grade(average_mark) # Calculate grade based on average mark # Output student details print("Student Name:", StudentName[i]) print("Combined Total Mark:", total_mark) print("Average Mark:", average_mark) print("Grade Awarded:", grade) print() # Empty line for readability
Solution:
# Sample data (already initialized) Account = [["John", "password1"], ["Alice", "password2"], ["Bob", "password3"]] AccDetails = [ [1000.0, 500.0, 200.0], [2000.0, 1000.0, 300.0], [1500.0, 800.0, 250.0] ] # Procedure to check if account ID, name, and password are correct def authenticate(account_id, name, password): if account_id < len(Account): if Account[account_id][0] == name and Account[account_id][1] == password: return True print("Authentication failed.") return False # Procedure to display balance def display_balance(account_id): print("Balance:", AccDetails[account_id][0]) # Procedure to withdraw money def withdraw_money(account_id, amount): balance, overdraft_limit, withdrawal_limit = AccDetails[account_id] if amount > balance + overdraft_limit: print("Withdrawal amount exceeds overdraft limit.") elif amount > withdrawal_limit: print("Withdrawal amount exceeds withdrawal limit.") else: AccDetails[account_id][0] -= amount print("Withdrawal successful.") # Procedure to deposit money def deposit_money(account_id, amount): AccDetails[account_id][0] += amount print("Deposit successful.") # Input: account ID, name, and password account_id = int(input("Enter account ID: ")) name = input("Enter your name: ") password = input("Enter your password: ") if authenticate(account_id, name, password): while True: print("Menu:") print("1. Display balance") print("2. Withdraw money") print("3. Deposit money") print("4. Exit") choice = input("Enter your choice: ") if choice == "1": display_balance(account_id) elif choice == "2": amount = float(input("Enter withdrawal amount: ")) withdraw_money(account_id, amount) elif choice == "3": amount = float(input("Enter deposit amount: ")) deposit_money(account_id, amount) elif choice == "4": print("Exiting...") break else: print("Invalid choice.")
Hint:
Account[0] = ["John", "password1"] AccDetails[0] = [1000.0, 500.0, 200.0]
Solution:
# Initialisation of Days array Days = { 1: "Sunday", 2: "Monday", 3: "Tuesday", 4: "Wednesday", 5: "Thursday", 6: "Friday", 7: "Saturday" } Readings = {} # Input temperatures in Readings array for R in range(1, 8): TotalDayTemp = 0 for C in range(1, 25): Temperature = float(input("Enter temperature {C} for {Days[R]}: ")) # Range check to ensure that temperature is between -20 and 50 while Temperature < -20.0 or Temperature > 50.0: print("Invalid value. Temperature must be between -20.0 and +50.0 inclusive. Enter temperature again: ") Temperature = float(input()) Readings[(R, C)] = Temperature TotalDayTemp += round(Temperature, 1) # Calculate average temperature for the day AverageTemp = round(TotalDayTemp / 24, 1) print("The average temperature on {Days[R]} was {AverageTemp} Celsius and {round(AverageTemp * 9 / 5 + 32, 1)} Fahrenheit") # Store the average temperature Days[R] = AverageTemp # Calculate average temperature for the week AverageWeekTemp = sum(Days.values()) / 7 print(f"The average temperature for the week was {AverageWeekTemp} Celsius and {round(AverageWeekTemp * 9 / 5 + 32, 1)} Fahrenheit")
Solution 1:
# Define the ranges for temperature and pulse readings TempLow = 31.6 TempHigh = 37.2 PulseLow = 55.0 PulseHigh = 100.0 # Sample data for Patient names and Readings Patient = ["John", "Alice", "Bob", "Emma"] # Sample patient names Readings = [ [35.5, 80], # Sample readings for patient 1 (John) [36.8, 95], # Sample readings for patient 2 (Alice) [37.5, 50], # Sample readings for patient 3 (Bob) [38.0, 105] # Sample readings for patient 4 (Emma) ] # Each sublist contains temperature and pulse readings for a patient # Define the CheckPatient procedure def CheckPatient(HospitalNumber): # Check if the hospital number is valid if HospitalNumber >= 1 and HospitalNumber <= 1000: # Output the patient's name print("Name of Patient:", Patient[HospitalNumber - 1]) # Check if both readings are within range if (Readings[HospitalNumber - 1][0] >= TempLow and Readings[HospitalNumber - 1][0] <= TempHigh) and (Readings[HospitalNumber - 1][1] >= PulseLow and Readings[HospitalNumber - 1][1] <= PulseHigh): print("Normal readings") # Check if one reading is out of range (temperature or pulse) elif (Readings[HospitalNumber - 1][0] < TempLow or Readings[HospitalNumber - 1][0] > TempHigh) and (Readings[HospitalNumber - 1][1] >= PulseLow and Readings[HospitalNumber - 1][1] <= PulseHigh): print("Warning: Temperature") elif (Readings[HospitalNumber - 1][0] >= TempLow and Readings[HospitalNumber - 1][0] <= TempHigh) and (Readings[HospitalNumber - 1][1] < PulseLow or Readings[HospitalNumber - 1][1] > PulseHigh): print("Warning: Pulse") # Check if both readings are out of range else: print("Severe warning: Pulse and Temperature") else: print("Hospital number not valid") # Test the procedure CheckPatient(1) # Sample test with hospital number 1
Solution-2
# Define the ranges for temperature and pulse readings TempLow = 31.6 TempHigh = 37.2 PulseLow = 55.0 PulseHigh = 100.0 # Sample data for Patient names and Readings Patient = ["John", "Alice", "Bob", "Emma"] # Sample patient names Readings = [ [35.5, 80], # Sample readings for patient 1 (John) [36.8, 95], # Sample readings for patient 2 (Alice) [37.5, 50], # Sample readings for patient 3 (Bob) [38.0, 105] # Sample readings for patient 4 (Emma) ] # Each sublist contains temperature and pulse readings for a patient # Define the CheckPatient procedure def CheckPatient(HospitalNumber): # Check if the hospital number is valid if 1 <= HospitalNumber <= 1000: # Get the patient's index in the arrays index = HospitalNumber - 1 # Output the patient's name print("Name of Patient:", Patient[index]) # Get the patient's readings temp_reading, pulse_reading = Readings[index] # Check if both readings are within range if TempLow <= temp_reading <= TempHigh and PulseLow <= pulse_reading <= PulseHigh: print("Normal readings") # Check if one reading is out of range (temperature or pulse) elif not (TempLow <= temp_reading <= TempHigh) and (PulseLow <= pulse_reading <= PulseHigh): print("Warning: Temperature") elif (TempLow <= temp_reading <= TempHigh) and not (PulseLow <= pulse_reading <= PulseHigh): print("Warning: Pulse") # Check if both readings are out of range else: print("Severe warning: Pulse and Temperature") else: print("Hospital number not valid") # Test the procedure CheckPatient(1) # Sample test with hospital number 1
# Define the baggage weight limits for different airlines airline_limits = { "Delta": 50, # Maximum baggage weight limit for Delta Airlines (in pounds) "American Airlines": 40, # Maximum baggage weight limit for American Airlines (in pounds) "United Airlines": 45 # Maximum baggage weight limit for United Airlines (in pounds) } # Sample data for passengers, their airlines, and baggage weights passenger_data = [ ["John", "Delta", 55], # John, Delta Airlines, 55 lbs ["Alice", "American Airlines", 38], # Alice, American Airlines, 38 lbs ["Bob", "United Airlines", 45], # Bob, United Airlines, 45 lbs ["Emma", "Delta", 60] # Emma, Delta Airlines, 60 lbs ] # Define the CheckBaggage procedure def CheckBaggage(passenger_data): for data in passenger_data: passenger_name, airline, baggage_weight = data if airline in airline_limits: max_limit = airline_limits[airline] if baggage_weight <= max_limit: print(passenger_name + "'s baggage weight is within the allowed limit (" + str(max_limit) + " lbs) for " + airline + ".") else: print(passenger_name + "'s baggage weight exceeds the allowed limit (" + str(max_limit) + " lbs) for " + airline + ".") else: print("Airline '" + airline + "' not found in the data for passenger " + passenger_name + ".") # Test the procedure CheckBaggage(passenger_data) # Test the procedure with sample passenger data